Friday, October 25, 2024
Mastering Laravel: Advanced Techniques for Building Scalable Applications
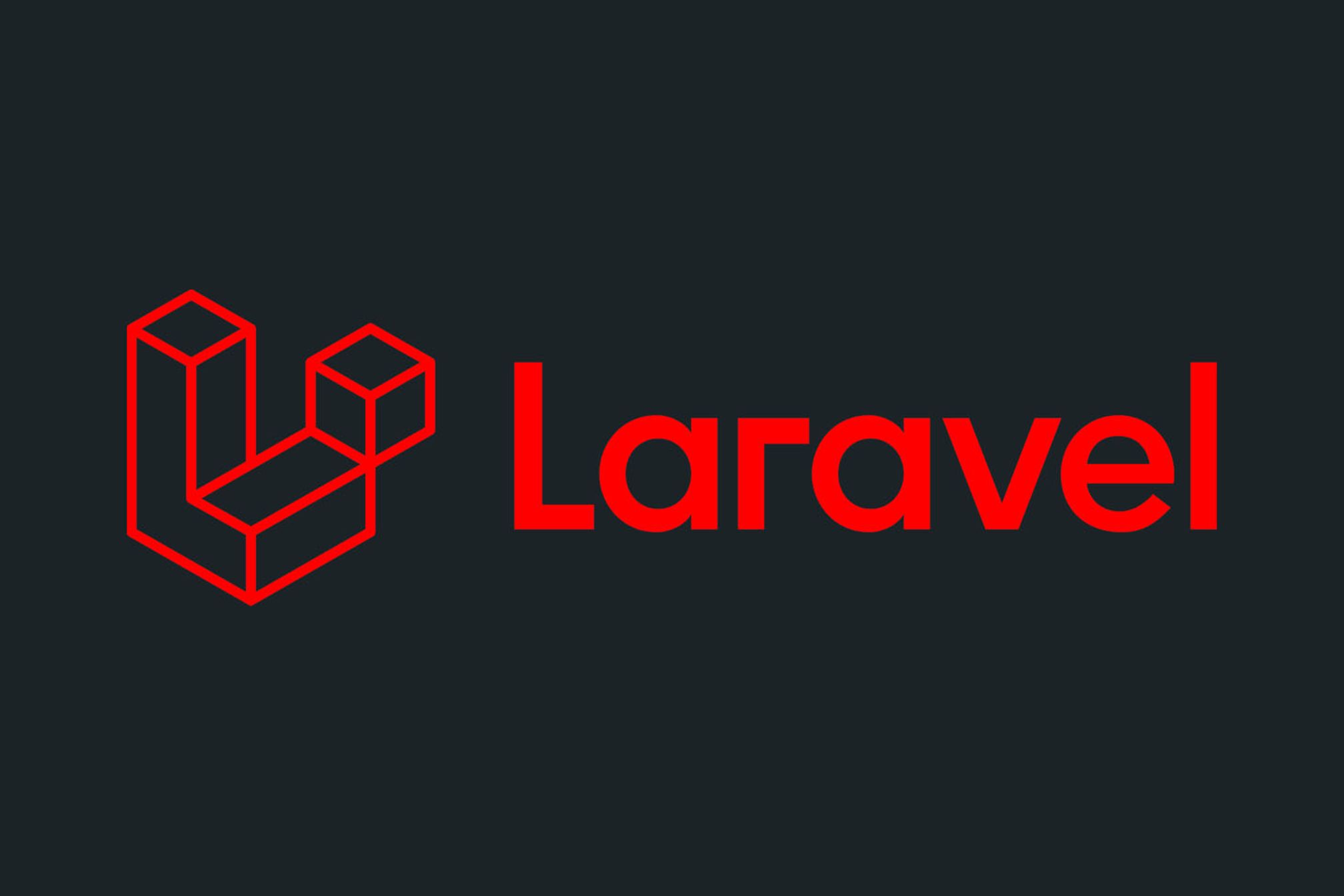
Introduction
Laravel is widely celebrated for its elegant syntax and developer-friendly features. However, to truly harness the power of Laravel, developers must go beyond the basics. In this post, we’ll explore advanced techniques that can help you build scalable and efficient applications using Laravel. We’ll cover service providers, custom facades, event-driven architecture, and more.
1. Utilizing Service Providers for Dependency Injection
Service providers are the backbone of Laravel's application bootstrapping. They are responsible for binding services into the service container, allowing you to easily manage dependencies throughout your application.
Example: Creating a Custom Service Provider
To create a custom service provider, you can use the Artisan command:
php artisan make:provider CustomServiceProvider
In your service provider, you can bind your classes:
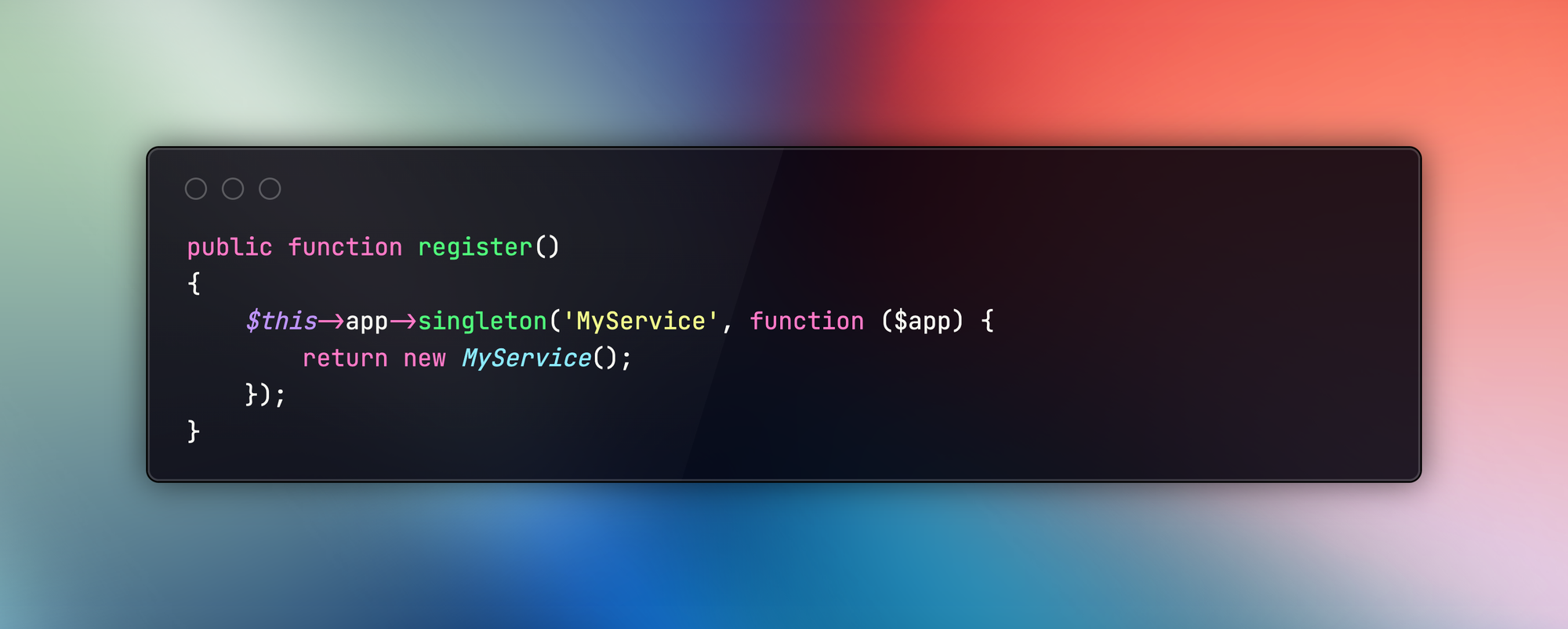
You can then inject MyService
anywhere in your application, promoting loose coupling and making testing easier.
2. Creating Custom Facades
Facades provide a static interface to classes that are available in the service container. They allow you to use classes easily without needing to resolve them out of the container each time.
Example: Creating a Custom Facade
First, create a class that you want to expose via a facade:
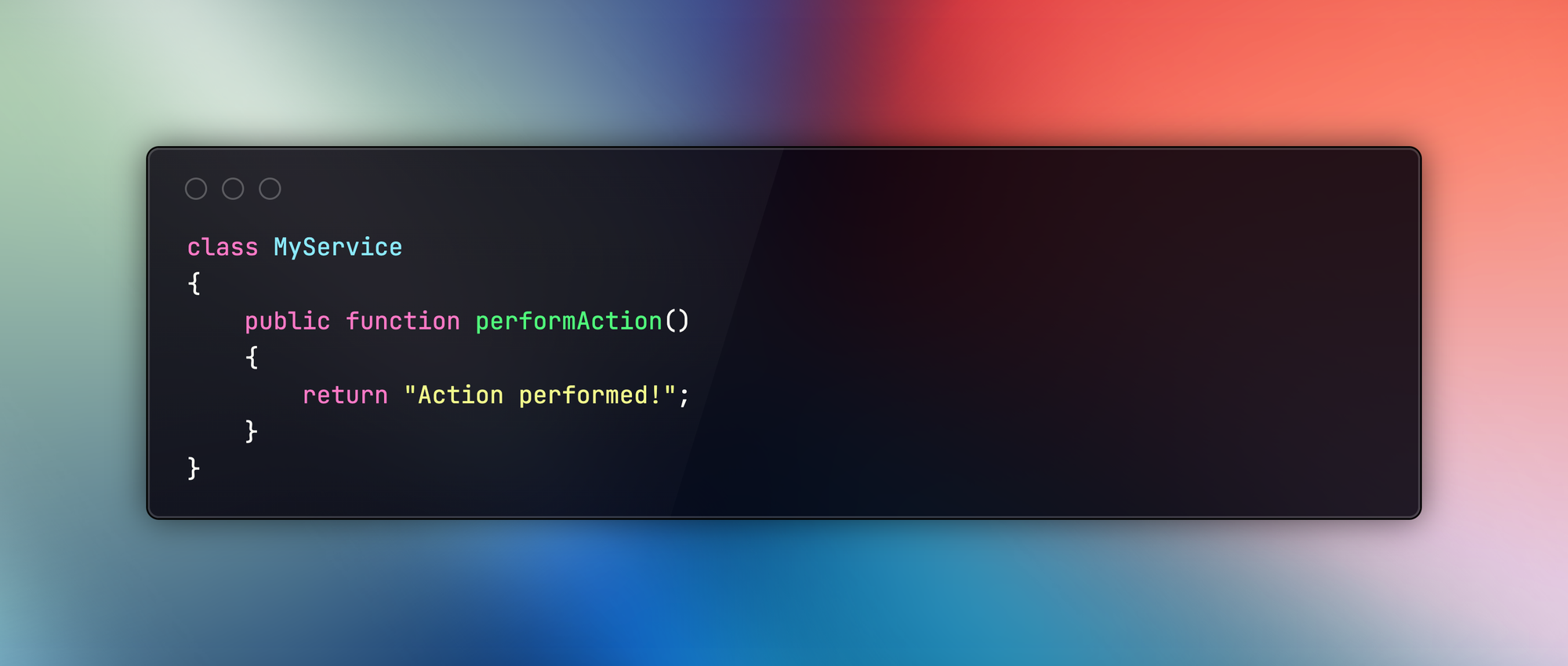
Then, create a facade class:
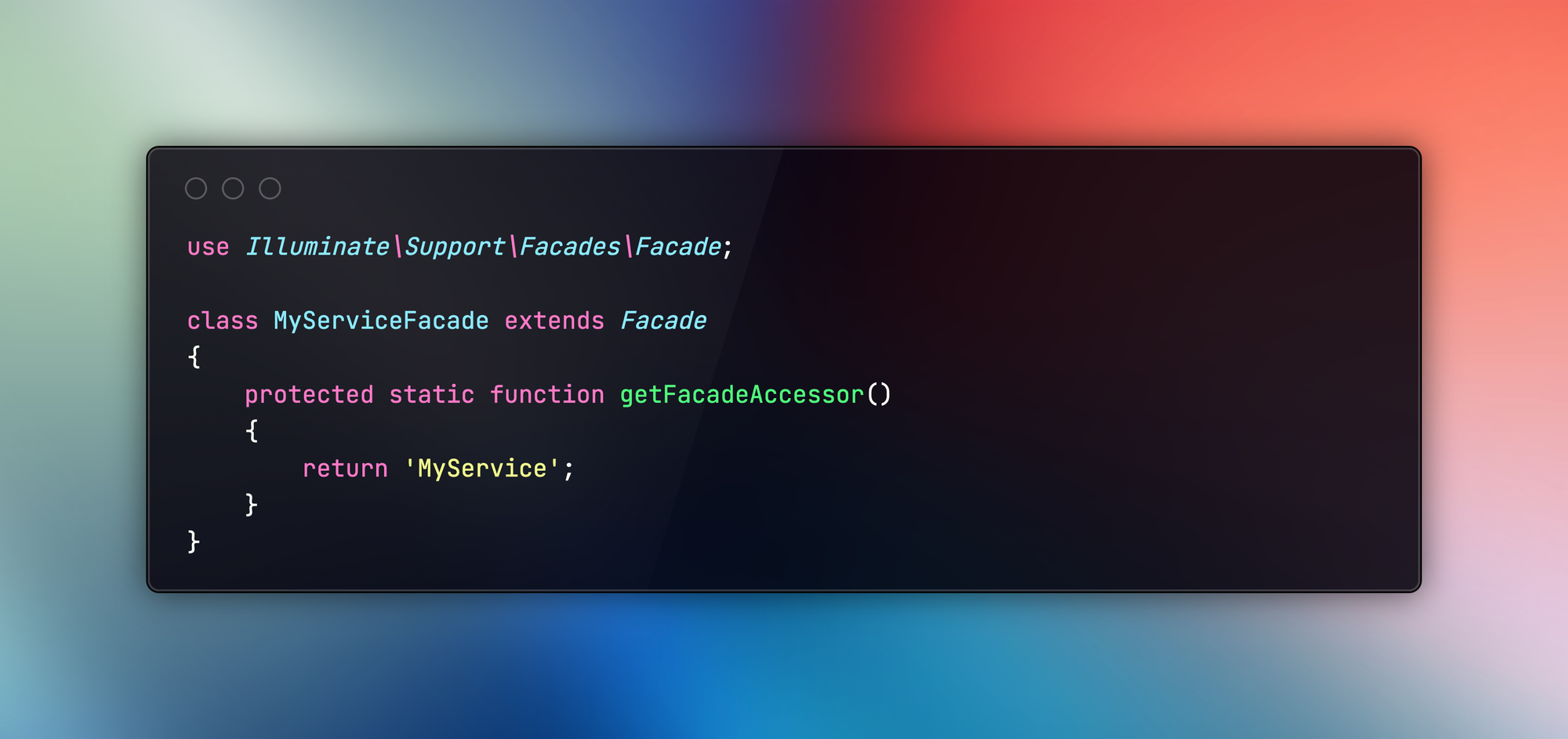
Now you can use MyServiceFacade
statically:
MyServiceFacade::performAction();
3. Implementing Event-Driven Architecture
Event-driven architecture allows your application to react to various actions in a decoupled manner. This approach can improve scalability and maintainability.
Example: Creating Events and Listeners
You can create an event using Artisan:
php artisan make:event UserRegistered
Then, create a listener:
php artisan make:listener SendWelcomeEmail
In your event, you can fire the event:
event(new UserRegistered($user));
And in your listener, handle the logic:
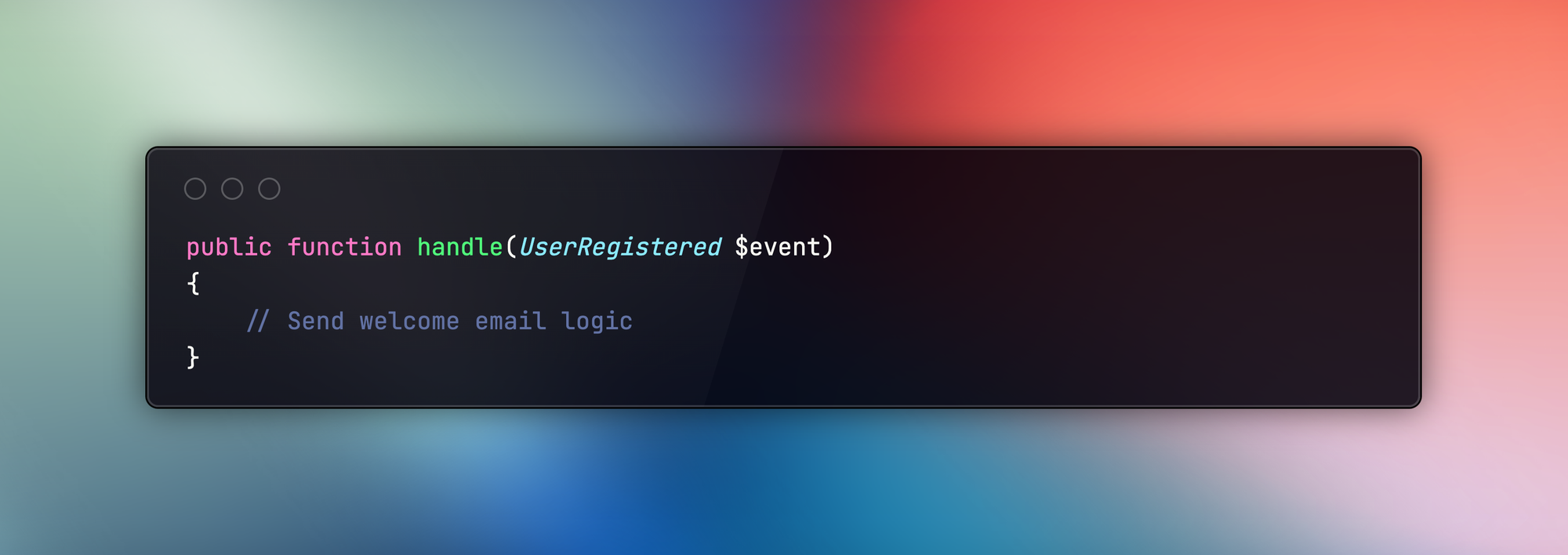
4. Advanced Query Techniques with Eloquent
Eloquent is powerful, but mastering advanced query techniques can significantly improve performance. Use scopes, eager loading, and chunking to optimize your queries.
Example: Using Scopes and Eager Loading
You can define scopes in your Eloquent models:
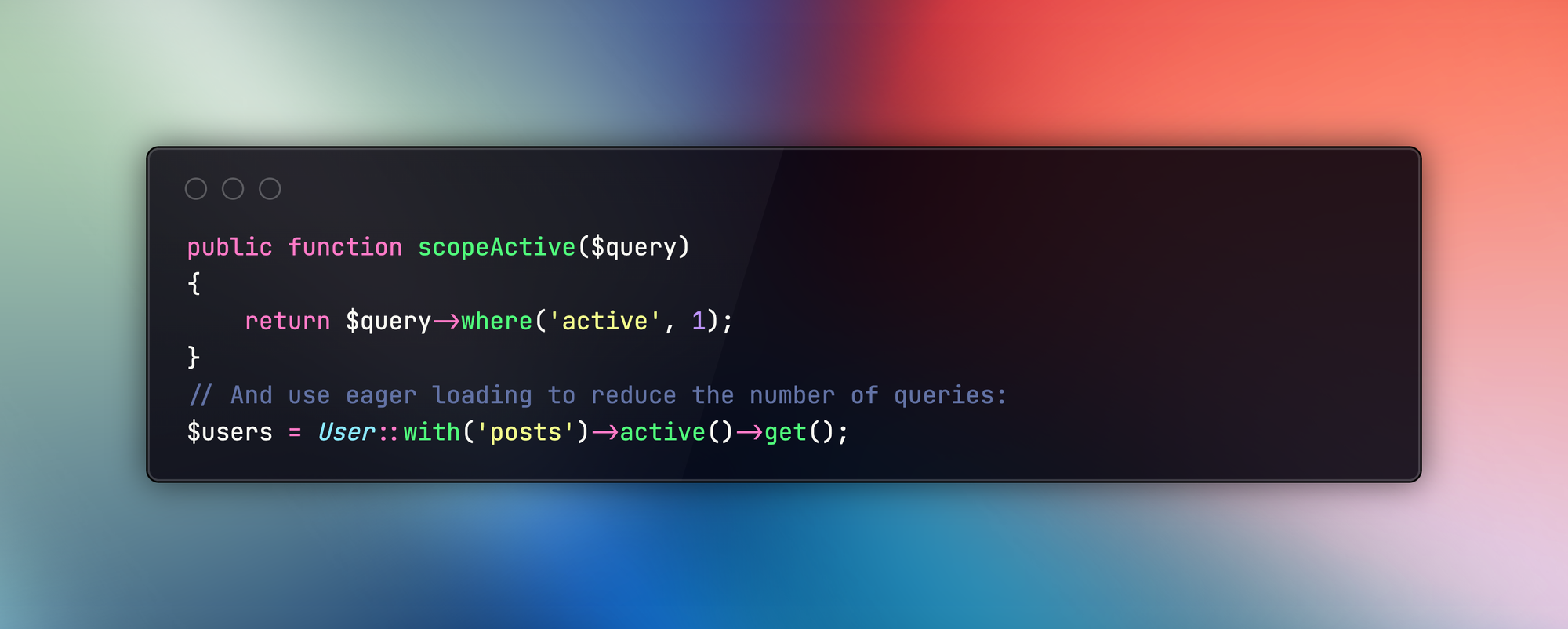
And use eager loading to reduce the number of queries:
$users = User::with('posts')->active()->get();
Conclusion
By leveraging these advanced techniques in Laravel, you can build applications that are not only efficient but also scalable and maintainable. Understanding service providers, creating custom facades, implementing event-driven architecture, and mastering Eloquent will elevate your development skills and allow you to take full advantage of what Laravel has to offer.